Download file có dung lượng lớn bằng ASP.NET
by My Love
21/07/2020, 2:45 PM | 21/07/2020, 2:45 PM | 1.3K | 020107
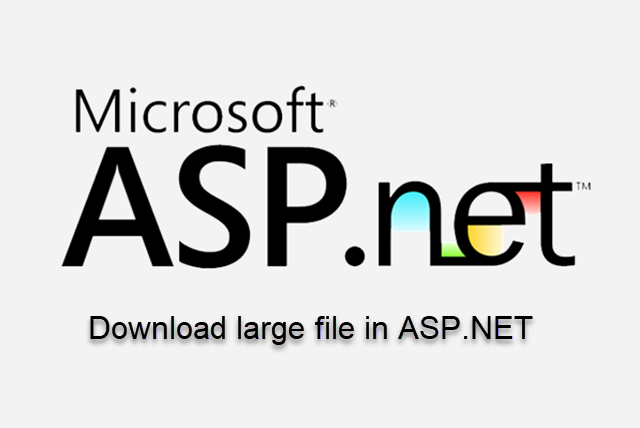
Khi làm việc trong ứng dụng ASP.NET chắc hẳn bạn không xa lạ gì với việc Upload và Download file, công việc này cũng tương đối đơn giản, để tải một file từ máy chủ server về bạn chỉ cần một đoạn code nhỏ như sau :
Response.ContentType = "application/octet-stream";
Response.AppendHeader("Content-Disposition", "attachment; filename=MyFile.rar");
Response.TransmitFile(Server.MapPath("~/Data/MyFile.rar"));
Response.End();
Tuy nhiên khi tải một file có dung lượng lớn thì sẽ gặp vấn đề, lúc này để tải file có dung lượng lớn nhiều GB thì bạn có thể sử dụng code sau :
Tạo một function download
public static void DownloadLargeFile(string DownloadFileName, string FilePath, string ContentType, HttpResponse response)
{
Stream stream = null;
int bufferSize = 1048576;
byte[] buffer = new Byte[bufferSize];
int length;
long lengthToRead;
try
{
stream = new FileStream(FilePath, FileMode.Open, FileAccess.Read, FileShare.Read);
lengthToRead = stream.Length;
response.ContentType = ContentType;
response.AddHeader("Content-Disposition", "attachment; filename=" + HttpUtility.UrlEncode(DownloadFileName, System.Text.Encoding.UTF8));
while (lengthToRead > 0)
{
if (response.IsClientConnected)
{
length = stream.Read(buffer, 0, bufferSize);
response.OutputStream.Write(buffer, 0, length);
response.Flush();
lengthToRead = lengthToRead - length;
}
else
{
lengthToRead = -1;
}
}
}
catch (Exception exp)
{
response.ContentType = "text/html";
response.Write("Error : " + exp.Message);
}
finally
{
if (stream != null)
{
stream.Close();
}
response.End();
response.Close();
}
}
Khi sử dụng chỉ cần gọi function trên :
DownloadLargeFile("MyFile.rar", Server.MapPath("~/Data/" + "MyFile.rar"), "APPLICATION/OCTET-STREAM", HttpContext.Current.Response);
- Ngoài ra bạn cũng có thể sử dụng đoạn code sau :
Response.ContentType = "application/octet-stream";
Response.AppendHeader("Content-Disposition", "attachment; filename=MyFile.rar");
const int bufferLength = 10000;
byte[] buffer = new Byte[bufferLength];
int length = 0;
Stream download = null;
try
{
download = new FileStream(Server.MapPath("~/Data/MyFile.rar"),FileMode.Open, FileAccess.Read);
do
{
if (Response.IsClientConnected)
{
length = download.Read(buffer, 0, bufferLength);
Response.OutputStream.Write(buffer, 0, length);
buffer = new Byte[bufferLength];
}
else
{
length = -1;
}
}
while (length > 0);
Response.Flush();
Response.End();
}
finally
{
if (download != null)
download.Close();
}
Chúc bạn thành công !