Lấy danh sách các bản cập nhật, lịch sử Windows Update bằng C#
by My Love
22/12/2019, 10:13 AM | 22/12/2019, 10:13 AM | 1.4K | 084
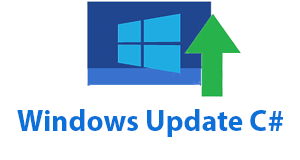
Trong môi trường Windows, để lấy xem các bản cập nhật Windows bạn có thể vào đường dẫn
Control Panel\Programs\Programs and Features\View Installed update. Hoặc dùng
Command Prompt hay Windows
PowerShell để lấy danh sách một cách dễ dàng. Tuy nhiên với lập trình viên trong một số trường hợp bạn cần lấy danh sách các bản Windows Update bằng ngôn ngữ
C# thì thế nào ? có 2 cách để lấy danh sách này.
Đầu tiên cần tạo một class lưu các thông tin cần lấy
WindowsUpdate.cs
public class WindowsUpdate
{
public string HotFixId { get; set; }
public string Description { get; set; }
public string InstalledOn { get; set; }
}
1. Sử dụng ManagementObjectSearcher
Trong Windows bạn có thể sử dụng lệnh
Get-HotFix trong Windows
PowerShell để lấy thông tin như sau :
Sử dụng lớp
ManagementObjectSearcher sẽ cho kết quả giống với lệnh
Get-HotFix trong
PowerShell. Với cách này bạn cần sử dụng thư viện
System.Management;
-Bước 1 : Add thư viện
System.Management; vào
Project
- Bước 2: Cần thêm thư viện
using System.Management;
- Bước 3 : Sử dụng đoạn code sau để lấy danh sách các bản
Windows Update
public void GetWindowsUpdate()
{
const string querys = "SELECT * FROM Win32_QuickFixEngineering";
//const string querys = "SELECT HotFixID FROM Win32_QuickFixEngineering"; // Chỉ lấy HotFixID
var search = new ManagementObjectSearcher(querys);
var collection = search.Get();
var listUpdate = new List<WindowsUpdate>();
foreach (ManagementObject quickfix in collection)
{
// lấy các trường HotFixID, Description, InstalledOn, có thể lấy các trường khác nếu muốn
string hotfix = quickfix["HotFixID"].ToString();
string Description = quickfix["Description"].ToString();
string InstalledOn = quickfix["InstalledOn"].ToString();
listUpdate.Add(new WindowsUpdate() { HotFixId = hotfix, Description = Description, InstalledOn = InstalledOn });
}
// đổ dữ liệu vào dataGridView
dataGridView1.DataSource = listUpdate;
}
2. Sử dụng thư viện WUApiLib
Cách này sử dụng thư viện
WUApiLib để lấy danh sách các bản cập nhật, kết quả trả về sẽ giống với
update history trong Windows
- Bước 1: Cần thêm thư viện
WUApiLib vào
Project
- Bước 2: Add thư viện using
WUApiLib;
- Bước 3: Sử dụng đoạn code sau :
public void GetUpdates()
{
var updateSession = new UpdateSession();
var updateSearcher = updateSession.CreateUpdateSearcher();
var count = updateSearcher.GetTotalHistoryCount();
var history = updateSearcher.QueryHistory(0, count);
var listUpdate = new List<WindowsUpdate>();
string lKB;
for (int i = 0; i < count; i++)
{
try
{
lKB = getKB_RegEx(history[i].Title);
}
catch (Exception)
{
lKB = string.Empty;
}
if (!String.IsNullOrEmpty(history[i].Title))
{
try
{
listUpdate.Add(new WindowsUpdate()
{
HotFixId = lKB,
InstalledOn = history[i].Date.ToString(),
Description = history[i].Description
});
}
catch (Exception)
{
}
}
}
// đổ dữ liệu vào dataGridView
dataGridView1.DataSource = listUpdate;
}
// getKB_RegEx và getKB_RegEx2 dùng để trả về định dạnh HotFixID dạng: KB947789
public string getKB_RegEx(string pTitle)
{
string lSerachPattern = @"(((\w{2}\d+) ?))";
string lInputString = pTitle;
string lResultString = string.Empty;
Regex lRegex = new Regex(lSerachPattern, RegexOptions.Multiline);
MatchCollection lMatchCollection = lRegex.Matches(lInputString);
if (lMatchCollection.Count > 0)
{
foreach (Match match in lMatchCollection)
lResultString = match.Value;
}
if (lResultString.StartsWith("KB", true, CultureInfo.InvariantCulture))
return lResultString; //normalfall, KB in runden klammen: Windows-Tool zum Entfernen bösartiger Software x64 - Mai 2018 (KB890830)
else
return getKB_RegEx2(pTitle); //Spezialfall, KB ohne runde Klammern: Definitionsupdate für Windows Defender Antivirus – KB2267602 (Definition 1.267.1832.0)
}
public string getKB_RegEx2(string pTitle)
{
string lSerachPattern = @"KB\d+ ?";
string lInputString = pTitle.ToUpper();
string lResultString = string.Empty;
Regex lRegex = new Regex(lSerachPattern, RegexOptions.Multiline);
MatchCollection lMatchCollection = lRegex.Matches(lInputString);
if (lMatchCollection.Count > 0)
{
foreach (Match match in lMatchCollection)
lResultString = match.Value;
}
return lResultString;
}
- Bạn sẽ cần add thêm một số thư viện khác như :
using System.Text.RegularExpressions;
using System.Collections.Generic;
using System.Globalization;
- Cách thứ 2 này bạn có thể tham khảo thêm tại
https://github.com/avogelba/GetUpdates